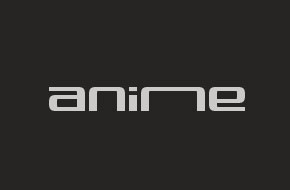
Expand Your Animation Toolkit with the Anime.js library
Surpass the limits of CSS with beautiful animations!
We’ve previously talked about creating your own CSS animations and also how to trigger them via javascript. This is great for adding life to your website, and drawing users’ attention to where you want it to go. In this post, we’ll be going over one of our favorite frameworks for improving and optimizing javascript animations: anime.js.
Why anime.js?
- It’s extremely lightweight, clocking in at 14kb minified and only 6kb gzipped (great for site loading speed).
- It runs entirely on vanilla javascript, which means it has no dependencies and it runs faster than jquery (while still remaining compatible with it).
- In addition to being able to work with all CSS properties, it can also animate SVGs and HTML attributes.
- As animations get more complex and more elements are being animated, anime.js allows you to put various animations together into an object that can be triggered at will.
Getting started with the library
This library is available as a node package module, via content delivery network (CDN) links, or downloading and enqueueing the files directly into your theme. (Not sure what these mean, or how to do them? Reach out to us for a free quote!)
After adding anime.js to your project, you can hook an element into the library to start animating it using code like the example below:
// The HTML
<div class='your-animation-class'></div>
// The Javascript
document.addEventListener('DOMContentLoaded', () => {
anime({
targets: '.your-animation-class',
duration: 1500,
easing: 'easeInOutQuad',
});
}
- targets: the selectors (HTML classes or IDs) for the element(s) we want to animate
- duration: time in milliseconds for the animation to run
- easing: how the animation starts and ends. You can have it run all the same speed, or have it “ease in” or “ease out” of the animation for a smoother effect.
Declaring it directly like this causes the animation to begin immediately, but nothing is happening since we haven’t added any CSS transitions yet! One of the benefits of this library is that you can include the CSS animation properties within the variable itself, keeping everything in one place. You can then call the ‘play’ method to trigger the animations.
let demo = anime({
targets: '.animation-demo',
duration: 1500,
easing: 'easeInOutQuad",
translateX: 250, // Creates a CSS property
scale: 1.3 // Creates a CSS property
});
Any CSS property will work as an animation parameter within the “anime” object. You can either append each property as an additional parameter pair within the object, or if you would prefer keyframe level control, you would use the keyframes parameter instead like how you write it in a CSS file:
// The CSS
keyframes: [
{translateX:50; scale:1.1},
{translateX:150; scale:1.2},
{translateX:250; scale:1.3}
]
The deeper benefits of anime.js
The animation values aren’t limited to hard values either. You can use function-based values or even anime’s built-in random number generator for an animation that’s different every time.
As your animations get more complex, you might want to use a timeline object, which allows you to set some ‘master’ parameters like duration and easing which you can then add build other animation objects off of:
let timeline = anime.timeline({});
demo.add({
targets: '.examples',
translateX: 50,
scale: 1.1
}, offset);
demo.play(); // Plays the animation
If you leave the “offset” value blank, the animations will play in the order they are added within the timeline. Otherwise, you can either give them an offset from the beginning of the timeline or from the end of the previous animation.
If an animation is targeting multiple elements and you would like their animations to be staggered, anime.js will do that out of the box for you too!
anime({
targets: '.examples',
translateX: 50,
scale: 1.3
delay: anime.stagger(100)
});
Additional Helpful Tips
Anime.js comes with a “seek” parameter which allows you to tie the animation progress to the user’s scrolling.
You can create your own pseudo animation library by creating common anime objects and timelines and putting them within a callback function that you can then pass target element selectors into.
const playDemo = (elem) => {
let demo = anime({
targets: elem,
translateX: 50,
scale: 1.3
});
}
With the above method combined with function-based values, you could create reasonably dynamic yet consistent in design animations throughout a website with minimal lines of code.
SVG support out of the box is also fantastic, though that is its own rabbit hole perhaps to be explored in a later blog post. For now, we’ll just give a brief overview of what anime can do here.
- Motion path: animating an element along a svg path
- Morphing: SVG objects can morph shapes as long as its number of points remain the same.
- Line drawing: animating the drawing of a svg path
Truly, your imagination is the limit when it comes to the front-end and capturing visual interest. We here at Mr. WPress are experts at making your vision come to life on the web. Need help with anime.js, just regular CSS animation, or anything else? You tell us what you want through a free quote, and we can build it. Easy as that!