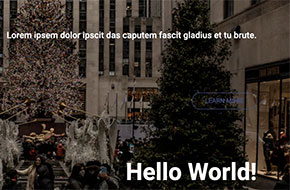
Revisiting Advanced Custom Fields post-Gutenberg
If you’ve been a longtime reader of our blog, you’ll remember our brief overview of Advanced Custom Fields and its ability to help us create dynamic templates with minimal end-user input. However, it’s been about 2 years since then and Gutenberg and various other page builders have ushered in a ‘Block Editor’ paradigm. This is where ACF Blocks comes in: providing an easy way for developers to tap into all the new functionality without necessarily having to learn advanced coding languages like React.
The Foundational Code
Today, we’ll be running you through the creation of a block. Let’s begin with adding this code into your functions.php:
add_action('acf/init', 'my_acf_init_block_types');
function my_acf_init_block_types() {
if (function_exists('acf_register_block_type')) {
acf_register_block_type([
'name' => 'page-hero',
'title' => __('Page Hero'),
'description' => __('A page image header block'),
'render_template' => 'block-templates/page-hero/page-hero.php',
'category' => 'custom',
'icon' => 'cover-image',
'keywords' => ['hero', 'header', 'image'],
'mode' => 'edit'
]);
}
}
This is an action hook function that’s called upon the initialization of the ACF plugin. It checks for the existence of the registration function before registering any blocks. The title of this block is “Page Hero”, and will be the foundation for creating an attractive banner at the top of whatever page we’d like. Saving it in a block like this allows us to add it quickly and effectively, even with a little bit of customization.
Block registration parameters:
In our function within the function to register a block type, there were several parameters defined. Here’s what they all mean:
- name: Advanced Custom Fields saves all custom blocks under the ‘acf/insert-block-name’ pattern into the database.
- title: What the block will be called in the editing dashboard.
- description: Blurb inserted into the block’s metabox sidebar header.
- render_template: I’ve chosen to render the block’s HTML via a template file but you can also use a callback function in which case you’d be using ‘render_callback’ instead.
- category: What the block will be grouped under
- icon: Chosen dashicon as part of block identifier
- keywords: with which to search the block for by
- mode: Whether the block appears as a field group (edit) or WYSIWYG (preview) in the editing dashboard.
The next step is to create your field group in the ‘Custom Fields’ page of your dashboard. This process is the same as is standard, except we now add a location rule attaching it to our newly created block.
The template file and additional features
/**
Display Custom ACF Block
*/
<div class="<?php echo $block['className']; ?>">
<img src="<?php the_field('page_hero_img'); ?>">
<p><?php the_field('page_hero_copy'); ?></p>
<a href="<?php echo get_field('page_hero_link')['url']; ?>">
<?php echo get_field('page_hero_link')['title']; ?>
</a>
<h1><?php the_title(); ?></h1>
</div>
Here’s the code to turn our foundational function into something that displays on the frontend of the site. We start with a comment section at the top designating what this file is for, just for organization’s sake. We recommend assigning your custom blocks with identifying classes for easier styling and debugging. You’ll notice that we’re checking for $block[‘className’]. That is because ACF blocks support almost all of the same features as core WordPress. Functionality around classes is enabled by default, but it’s all controlled by the ‘supports’ parameter. Let’s add the following to our acf_register_block_type() function from the first section.
'supports' => [
'anchor' => true,
'align' => true,
'color' => [
'text' => true,
'background' => false,
'gradient' => false
]
]
These are some of the most helpful extra parameters:
- anchor: enables block ID and therefore links to said element
- align: allows user to select the alignment of block
- color: allows user to select colors (of theme) of block elements
For any style-based properties that are enabled, we can call upon the user-selected values within the template as $block[propertyName]. (Note: make sure to use camelCase! i.e. text-color => $block[textColor]). We typically store these in various classes or data-attributes within the block depending on what we need the block to do.
This is how the block looks in the page editor. You can see all our settings are reflected here: from the icon and block description to the enabled ‘Color’ and ‘Advanced’ options. (For colors, only text color was enabled and thus it’s all you’ll see).
Styling the Custom Block
Finally, we are on to styling. ACF allows you to enqueue block-specific assets with any of the following 3 parameters in acf_register_block_type():
- ‘enqueue_styles’ for css files only
- ‘enqueue_scripts’ for js files only
- ‘enqueue_assets’ for both.
Alternatively, you could also insert inline or tags directly into the template. These resources will be run any and every time the corresponding block appears on the page. If you expect multiple instances of the same block on the same page, we recommend to conditionally enqueue the assets based on whether the page has the block in question. Essentially like so:
if ((is_single() || is_page()) && has_block('acf/page-hero') {
// enqueue a script or style or other code to run
}
Either way, this allows you to only load the necessary resources when the block is present on a given page making the overall site more efficient and performant.
The finished product
The styling can be expanded upon using CSS, but this is a simple and effective example that shows all the features available at your disposal.
While base Advanced Custom Fields is free to use, ACF Blocks is limited to the Pro version of the plugin. We believe in its utility and our clients love being able to use a more intuitive and visual editor.
Need help getting started with Advanced Custom Fields, or anything on your website? Don’t hesitate to reach out for a free quote! We’re WordPress experts at Mr. WPress, and that includes custom post types and fields. We want to help you create the perfect website!