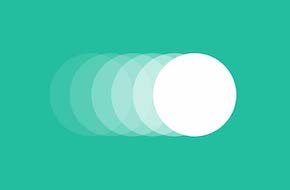
Foundational JavaScript to trigger CSS animations
Some short functions can go a long way!
In our previous post on CSS animations, we walked through how to create custom CSS animations using the wide capabilities of the language. However, we only touched briefly on how to actually trigger these animations. When using the animation property in CSS, the animation triggers right away (or after a short, configured delay). But even with a delay, CSS doesn’t come with the ability to tell when an element enters the screen. Let’s say you set a delay for 1 minute, but by the time one minute passes any given user may not have reached the animating element, or may have passed it entirely.
One potential workaround here is to have the animation loop indefinitely, but this isn’t considered user-friendly. The user might discover the element in the middle of an animation, causing confusion. Or they may find the animation distracting, with no way to stop it. Best practices generally say to only allow 3 loops of any given animation or motion effect.
So how do we reliably and responsibly trigger animations? The answer is JavaScript, and jQuery in particular.
First step: set up the function to add the class
First thing’s first is to get our CSS ready. Test out your animation and set it to apply on a given class (potentially named after the animation, though anything will work). Then, when we set up our jQuery trigger, we’ll have the trigger add the class to the HTML element we want to animate. When the class is added, the animation will trigger as if it first loaded in. Associating the animation to a class like this is particularily handy, because it allows us to enable the animation on all kinds of elements throughout a page or even the whole site, with only CSS declaration.
With this in mind, our jQuery function body will look something like this:
$('.target-element').addClass('animate');
The first pair of parenthesis after the dollar sign is the target, and then after the period, we tell it to add the class of animate. But this is just the code of how to add the class, we need to set up the function that triggers it.
Trigger the animation on load
Even though a CSS animation attribute will trigger on load, this still isn’t always the best practice. It’s possible that certain elements load in before others, causing potentially confusing or even totally missable animations. You spent the time to create these awesome custom effects, you want to make sure they’re appreciated!
So even if you only want the animation to trigger on load, consider using the .ready or .load events in jQuery. Depending on how you set it up, this can wait for a certain element, or the entire page to be ready before the function triggers. If we wanted to wait for the whole page to be ready, and then trigger the animation, the jQuery function would look like this:
jQuery(function($) {
$(document).ready(function() {
$('.target-element').addClass('animate');
});
});
This is a very simple jQuery functions that triggers the action discussed in the prior section when the entire page (or “document”) is fully loaded and ready for the user to interact with it. Notice we wrapped the entire function inside of a jQuery function – this just ensures that the jQuery framework and the necessary $ variable are loaded in properly for our usage.
Do something when the user hovers
Both jQuery or CSS – differences
Another common animation trigger is when the user hovers over an element. This one is actually possible in CSS alone, using the :hover
state. However, this does have some limitations built in. For one, this can only cause an animation to trigger on the selected element or a child of said element. If you wanted to hover a button in a div that wasn’t attached to the actual element you wanted to animate, CSS wouldn’t cut it. This also is restricted to when the element in question is being actively hovered. If the user stops hovering, the animation abruptly ends as well.
But with jQuery, these issues are nonexistent. You can hover any element and trigger the animation anywhere else. And the animation will run its course even if the user stops hovering. No abrupt stops or sudden jumps. The jQuery for hovering looks something like this:
jQuery(function($) {
$('.animation-trigger').hover(function() {
$('.target-element').addClass('animate'), ''
});
});
Note the comma with the empty quotes after the sub-function that adds the animate class. The jQuery hover method actually supports an action for both when the mouse enters the area of the targetted element, and for when it leaves. In this case, we don’t want to do anything when the user’s mouse leaves the element, though we’ll cover later potentially removing the class so the animation can be triggered again (preferably after a delay so that the first animation finishes first).
Listen for a click, and then trigger the animation
Similar to hover, the animation can be triggered whenver another element is clicked. Though unlike hover, this one is slightly simpler because it does not have two different states (mouse in and mouse out, of hover). But the overall structure is largely the same:
jQuery(function($) {
$('.animation-trigger').click(function() {
$('.target-element').addClass('animate');
});
});
Easy enough! Another example is…
Wait for the user to scroll to a certain element
More automated than click, you can wait to trigger the animation until the user scrolls to a specified element. This generally triggers as soon as the top of the element enters the screen, so consider setting to a separate element lower down, setting a delay, or setting an offset if you feel comfortable taking it up a level.
jQuery(function($) {
$('.animation-trigger').scroll(function() {
$('.target-element').addClass('animate');
});
});
Repeat the animation by toggling the class with another trigger
With all this knowledge, we can put in reverse to remove that animate class using these same triggers! We mentioned this when using hover already, but you could use a button that toggles the animation on and off, or set it to remove the animation class when scrolling far enough past the element to another one. This way, when the first even fires again, it will have a class to add that will trigger the animation. If you try to add the class when it already exists, nothing will happen. But if the class is gone, then it will trigger all over again!
One handy way to do this is by using a slightly different method call in your function:
jQuery(function($) {
$('.animation-trigger').click(function() {
$('.target-element').toggleClass('animate');
});
});
The only change here was we switched out “addClass” with “toggleClass.” As you might expect, this checks to see if the element has the class or not yet, and then switches states. If it doesn’t have the class, it will add it, and trigger the animation. If it does have the class already, it will instead remove it, priming the trigger to fire again.
Need help putting together a custom CSS animation, or finding the perfect trigger in jQuery? Don’t hestiate to reach out to us at Mr. WPress for a free quote! We’d be delighted to help you create your dream website with engaging and exciting animations.