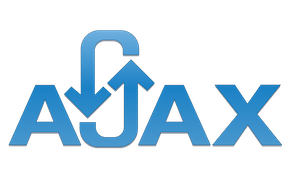
Submit and change information without refreshing the page
Create a smooth and dynamic user experience with AJAX
AJAX is a powerful tool that allows you to essentially connect JavaScript and PHP, having them share and change information without ever reloading the page. You can submit forms without changing pages, query the database to dynamically filter a list of posts, and so much more. This blog post will cover more of the general ideas of how AJAX works and when to use it, but if you’re interested in setting up AJAX for yourself, you can find tons of resources with code snippets and tutorials.
Configure the actions and callbacks for AJAX
A standard HTML form submission relies on the POST method, essentially sending variables from one URL to another. The variables are defined by the form fields, and then handled at the destination URL, whether they’re just being stored or creating dynamic content based on the response. AJAX essentially catches this submission before the destination URL is called, and handles all the variables in JavaScript, which can run without causing a page refresh.
With that in mind, the very first thing to do is set up the infrastructure to make these AJAX calls. Whether in a custom plugin or just the functions.php file, you’ll need to define and configure a few settings to set up where these calls should go. It’s also generally best practice to keep your AJAX scripts in a separate file, which will then need to be enqueued on the site in general (or at least the specific pages you need it to run).
Watch for triggers in the JS
To get going with AJAx functionality, you’ll need something to happen on the page. This is where JavaScript events come into play, and there’s nothing fancy about them yet. These are all the standard “ready”, “click”, and “submit” events, or anything else you’d like to watch for. Maybe you want something to happen when an input is just changed, that’s doable too. As long as you can watch for it in JavaScript, you can use it to start an AJAX call.
On some events, like form submissions, you may want to prevent the default behavior, but that can be achieved with a single line of code. Then you’ll loop through the HTML to grab all the data you need, put them into variables, and send them back in a special POST format via the settings you configured in the section above. The AJAX call has a specific functionality that doesn’t refresh the page like a normal POST functionality, but instead runs all on the same page without reloading.
Receive the response back in PHP
One of the elements you set up right at the start is a callback function, which is basically what handles the response from the AJAX script back in the PHP. The JavaScript sends over all the variables you’ve defined, and now you can use them in PHP to make changes beyond just the frontend. Generally, the first variable you’ll define in the JavaScript is the “task,” and then in PHP you’ll check that task variable and do different things depending on that task.
What you do, again, is built right into PHP. Just treat it like you’re submitting a form. Want to add rows to the database? Update a value? All of that is perfectly fine.
When that’s done, you need to prepare a response to send back to the JavaScript. Sometimes this can be as simple as a message that just says “Success.” Sometimes it may be multiple elements, for instance, both a text message and a URL so you can add a button. Sometimes it may be a full-fledged HTML template, which is perfectly fine too, depending on what kind of response you want your users to see.
For instance, if you’re using AJAX to run a custom filter on some posts, a good tactic is to create a shortcode that loops through the posts as a starting point. Then add a parameter to that shortcode based on filter criteria. Then, in the PHP processing after an AJAX call, you can pass the filter to the PHP, which can then be put into that shortcode, and the output of the shortcode can be put into a variable to pass back to the JavaScript.
Measure success back in the JS
With your response put together in the PHP, it’s time to return to the JavaScript to actually output it. Again, this can be as simple as defining a message banner and adding a simple success message inside. Or it could be changing the entire HTML of a container with filtered or otherwise altered content. All it takes is a simple .val() or .html() function, and you’ve got the setup to dynamically process any kind of action without ever reloading the page.
There are a few other conditions that are recommended to set up. Everything’s not always going to run perfectly, so it’s good to set up a failure case and message as well to ensure a smooth user experience and enable more effective debugging. This requires some additional code in both the JavaScript and PHP, but it follows the same general structure as the rest of the AJAX functionality.
Need help?
Need help getting started on your own AJAX project, or want to see some more practical examples? Don’t hesitate to reach out to us for a free quote! We’d be delighted to help you turn your website dream into a reality.